Concurrency in Golang
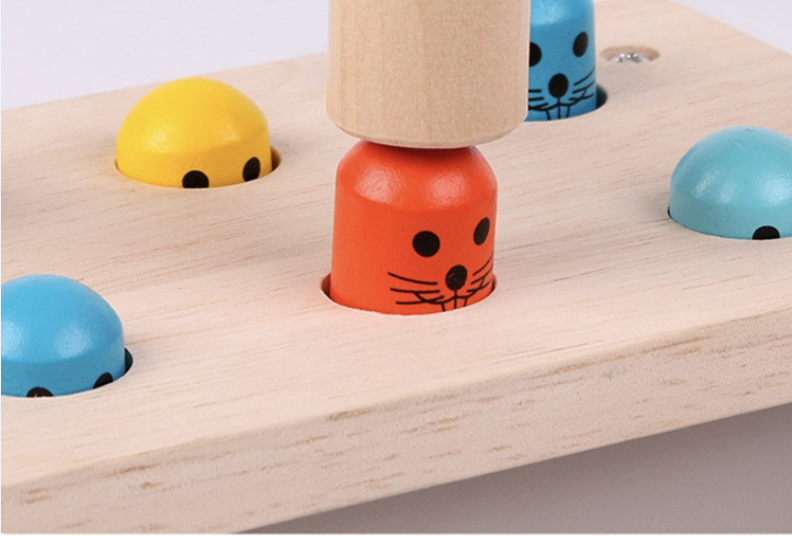
In this post we briefly looked at сoncurrency in general. Let's consider this topic for Golang. Specifically, there are two core features that make Go stand out: Goroutines and Channels. Combined, goroutines and channels are Go's take on concurrency.
Channels
Later we'll talk about Goroutines more. For now, simply think of them as threads and to run an function as goroutine you should use the operator "go" before the function call.
go myAwesomeFunction()
So, let's focus on channels. A channel is used to communicate between goroutines. They can be used to signal or pass data around. And by manage this communication - we can manage our сoncurrency processing.
// initilize a channel with integer type
myAwesomeChannel := make(chan int)
// send data to the channel
myAwesomeChannel <- 7
// read data from the channel
x := <- myAwesomeChannel
But why we need to manage our threads at all?
1) First of all, for keep their lifetime properly.
Let's consider next example -
package main
import "fmt"
func main() {
go squareNumbers(1, 6)
fmt.Println("Processed")
}
func squareNumbers(from int, to int) {
for i := from; i <= to; i++{
result := i * i
fmt.Println(i, " - ", result)
}
}
We have a simple function square(i) that prints square root of the given number and calling сoncurrency from our main() function for numbers 1 - 6. What do we expect?
1 - 5
2 - 4
3 - 9
4 - 16
5 - 25
6 - 36
Processed
But it is not so... in the result we can got next -
Processed
// or
1 - 5
3 - 9
6 - 36
Processed
After calling go square(i), main() function starts a goroutine, which starts executing in its context, independently of main() function. That is, in fact, main() and square(i) are executed in parallel. However, the main goroutine is the main function call. And if this function completes, then the execution of the entire program ends. Since calls to square(i) function represent goroutine, main() function does not wait for it to complete and continues executing after it is launched. Some code inside a goroutine may be unfinish before main() function, and accordingly, we will be able to see this result on the console. But a situation may arise where main() function is executed before calls to square(i) function. The logical solution - we should make main() wait until whole our goroutine will be completed.
For this, we can use our channel's read operator - <- channel. It has the ability to stop/blocked next code execution, util where will be any value.
package main
import "fmt"
func main() {
ch := make(chan bool)
go squareNumbers(1, 6)
<- ch // yeah, we can read a channel without set a result to var
fmt.Println("Processed")
}
func squareNumbers(from int, to int) {
for i := from; i <= to; i++{
result := i * i
fmt.Println(i, " - ", result)
}
}
The result -
6 - 36
3 - 9
1 - 1
2 - 4
5 - 25
4 - 16
fatal error: all goroutines are asleep - deadlock!
Looks like our squareNumbers() function has been completed! 🎉
BUT, where is "Processed" message? What is an error - deadlock?
2) Second need of manage threads is avoiding deadlocks.
Deadlock is when a group of goroutines are all blocking so none of them can continue. This is a common bug that you need to watch out for in concurrent programming.
In our code, main() function could not complete because we use the read channel operation - <- ch which blocked next code execution and all our program could not complete correctly.
So, let's try to use our channel's write operator - channel <- value. It has two properties: send value to a channel and stop/blocked next code execution, util another goroutine is ready to receive the value. Another goroutine in our case is main() function.
package main
import "fmt"
func main() {
ch := make(chan bool)
go squareNumbers(1, 6, ch)
<- ch // read channel, waiting any data
fmt.Println("Processed")
}
func squareNumbers(from int, to int, ch chan bool) {
for i := from; i <= to; i++{
result := i * i
fmt.Println(i, " - ", result)
}
ch <- true // write to channel
}
The result -
1 - 1
2 - 4
3 - 9
4 - 16
5 - 25
6 - 36
Processed
Well, we've fixed our deadlock issue! 🎉
Ok, let's stop "to beat around the bush" and get acquainted with other useful channel features.
Buffered Channels
Buffered channels are also created using the make() function, but the buffer(capacity) of the channel is passed to the function as the second argument.
myAwesomeChannel := make(chan int, 10)
A buffer allows the channel to hold a fixed number of values before sending blocks. This means writing on a buffered channel only blocks when the buffer is full, and receiving from a buffered channel only blocks only when the buffer is empty.
Please have a look next examples -
package main
import "fmt"
func main() {
ch := make(chan int, 3)
// write to channel
ch <- 2
ch <- 5
ch <- 87
// read from channel
fmt.Println(<-ch)
fmt.Println(<-ch)
fmt.Println(<-ch)
fmt.Println("Processed")
}
-------------
2
5
87
Processed
package main
import "fmt"
func main() {
ch := make(chan int, 3)
ch <- 2
ch <- 5
ch <- 87
ch <- 10 // locked - main() is waiting a free space in channel
fmt.Println("Processed")
}
-------------
fatal error: all goroutines are asleep - deadlock!
Closing channel
Once initialized, the channel is ready to transmit data. It is in an open state and we can interact with it until it is closed using the built-in close() function.
package main
import "fmt"
func main() {
ch := make(chan int, 3)
ch <- 2
ch <- 5
close(ch)
// ch <- 87 // error - the channel already closed
fmt.Println(<-ch) // 2
fmt.Println(<-ch) // 5
fmt.Println(<-ch) // 0
}
Writting on a closed channel will cause a panic. A panic on the main goroutine will cause the entire program to crash, and a panic in any other goroutine will cause that goroutine to crash. To avoid this we should check if a channel is closed -
v, ok := <-ch
if ok {
// channel opened and we can write to this
} else {
// channel closed. don't write to here
}
Closing isn't necessary. There's nothing wrong with leaving channels open, they'll still be garbage collected if they're unused. You should close channels to indicate explicitly to a receiver that nothing else is going to come across.
Synchronization via channel
Using channel gives us the ability to synchronize between different goroutines. For example, one goroutine performs some action, the result of which is used in another goroutine. Like in our Square Numbers program, so let's try use channel for synchronization.
package main
import "fmt"
func main() {
results := make(map[int] int) // init empty map to fill it next
ch := make(chan struct{}) // init "dummy" channel just for signaling
go squareNumbers(1, 6, ch, results)
<- ch // read channel, waiting closed
for i, v := range results {
fmt.Println(i, " - ", v)
}
fmt.Println("Processed")
}
func squareNumbers(from int, to int, c chan struct{}, res map[int] int) {
for i := from; i <= to; i++ {
res[i] = i * i // put the square result to map
}
close(c) // close the channel when our goroutine has completed
}
The result -
3 - 9
4 - 16
5 - 25
6 - 36
1 - 1
2 - 4
Processed
Looks like we resolved our issue. But what about sorting of results? Of course we can sort resulting map, but let's consider next channel trick.
Streaming data over channel
Often, one goroutine broadcasts to another goroutine via a channel not single values, but some data stream. In this case, the general algorithm is that the sender goroutine write data for some period. When the data to write is finished, the work is done, the sender closes the channel. For read values from a channel, we can use the same form of for loop that we use to iterate over arrays.
package main
import "fmt"
func main(){
ch := make(chan string)
go squareNumbers(1, 6, ch)
for res := range ch {
fmt.Println(res)
}
fmt.Println("Processed")
}
func squareNumbers(from int, to int, c chan string) {
for i := from; i <= to; i++ {
res := i * i // put the square result to map
c <- fmt.Sprintf("%v - %v", i, res)
}
close(c)
}
Final result -
1 - 1
2 - 4
3 - 9
4 - 16
5 - 25
6 - 36
Processed
Сonclusion
Of course, we have considered only base things about manage concurrency in Go using channels and pretty simple case for use that. I highly recommended to check this article to look many channel use cases.